A Leap Towards Expressive Coding With Record Patterns In Java 21
Published on 02 Nov 2023
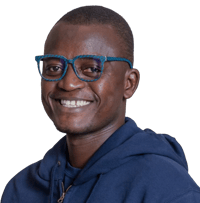
Java's journey towards fostering more expressive and efficient code has taken a remarkable leap with the advent of Record Patterns, encapsulated in JEP 440. This feature, previewed in both Java 19 and Java 20, is now a part of the language from Java 21, marking a significant stride towards reducing the verbosity traditionally associated with Java language. In this post, we will delve into the essence of Record Patterns, take a look at its benefits, and explore how it fits in with the existing pattern matching capabilities of Java.
Understanding Record Patterns
Record Patterns are a novel addition to Java's pattern matching constructs, extending its reach to de-structure instances of record classes. This enables more sophisticated data queries, allowing you to break down record values and nest record and type patterns for powerful, expressive, and modular data navigation and processing.
Simplifying Decision Choices
Consider a record representing a Person with a name and age.
public record Person(String name, int age) {
}
With Record Patterns, accessing the data becomes a breeze.
Person person = new Person("John Doe", 25);
if (person instanceof Person(var name, var age)) {
System.out.println("Name: " + name + ", Age: " + age);
}
Here, the instanceof operator alongside the Record Pattern Person(var name, var age) deconstructs the person object, providing direct access to its fields.
Where Record Patterns shine is when dealing with nested decision structures. Consider a scenario with nested records representing geometric shapes.
public sealed interface Shape permits Circle, Rectangle {
}
public record Circle(Point center, int radius) implements Shape {
}
public record Rectangle(Point topLeft, Point bottomRight) implements Shape {
}
public record Point(int x, int y) {
}
To calculate area based on the shape type:
void main() {
Shape shape = new Rectangle(new Point(0, 0), new Point(10, 5));
var area = calculateArea(shape);
System.out.println("Area: " + area);
}
public static double calculateArea(Shape shape) {
return switch (shape) {
case Rectangle(var topLeft, var bottomRight) -> (bottomRight.x() - topLeft.x()) * (bottomRight.y() - topLeft.y());
case Circle(_, var radius) -> Math.PI * radius * radius;
default -> throw new IllegalStateException("Unknown shape: " + shape);
};
}
In this example, we define a method calculateArea
which uses a switch
expression to destructure the Shape
object into its specific types (Rectangle
or Circle
) and calculates the area accordingly. In the Rectangle
case, it extracts the top-left and bottom-right points, and in the Circle
case, it extracts the radius while ignoring the center point using the _
wildcard.
Conclusion
JEP 440 has undoubtedly enriched the Java programming language, offering a robust construct to navigate complex decision choices. The ability to destructure records and nest patterns ushers the Java language into a realm of more expressive and less boilerplate code. As Java continues its stride towards modernization, features like Record Patterns play a pivotal role in ensuring the language remains expressive and enjoyable to work with. I for one, am very excited about all the code readability enhancements in Java 21.
Found this interesting? Try some of our other guides:
- Application Porting 101: How to Seamlessly Transition to Cloud Services
- No Nonsense Guide to JVM Implementations: OpenJDK, OpenJ9, GraalVM
- How to Identify Issues and Improve Application Performance
Fully managed Jakarta EE Deployment. ✅Handles Kubernetes for you.✅ 15 day free trial available.✅
Payara Cloud.
Related Posts
Virtual Payara Conference is this week! See the Agenda Highlights
Published on 09 Dec 2024
by Dominika Tasarz
0 Comments
Virtual Payara Conference - find out more and register here - is a two-day, free event that brings together industry leaders, developers, and innovators to explore the latest advancements and strategic insights in the world of Java and Jakarta ...
Join us for the London Java Community Unconference 2024
Published on 21 Nov 2024
by Dominika Tasarz
0 Comments