Nugget Friday - Streamlining Configuration with MicroProfile Config
Published on 27 Sep 2024
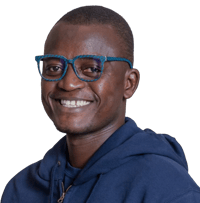
Managing configurations in enterprise Java application can often feel like a daunting task. As applications scale, configuration values become scattered across multiple files, formats, and environments—creating complexity and increasing the risk of errors. From juggling different settings for development, testing and production environments to dealing with the frustration of restarting applications for minor config changes, these challenges slow down development and impact productivity.
But what if there was a better way to manage configurations that are flexible, dynamic and type-safe? This Nugget Friday, we discuss MicroProfile Config—a powerful, standards-based solution designed to simplify configuration management for Java EE and Jakarta EE applications.
The Problem
Configuration management in non-trivial enterprise Java applications has long presented a range of challenges, including:
- Scattered Configuration: Configuration values are spread across multiple files and formats (properties files, XML, environment variables, etc.), leading to fragmented management.
- Environment-Specific Settings: Managing separate configurations for development, testing, staging, and production environments is complex and error-prone.
- Runtime Changes: A restart to apply new configuration values is often necessary, disrupting application uptime.
- Type Safety: The lack of type-safe configuration access can lead to potential runtime errors.
- Modularity: Organizing configurations for different components within a large application can be difficult.
For example, consider a traditional approach that involves reading from a properties file:
public class DatabaseConfig {
public static String getDbUrl() {
Properties props = new Properties();
try (InputStream is = DatabaseConfig.class.getResourceAsStream("/config.properties")) {
props.load(is);
} catch (IOException e) {
throw new RuntimeException("Failed to load config", e);
}
return props.getProperty("db.url");
}
}
This approach is prone to errors, not type-safe and doesn't easily support multiple configuration sources or dynamic updates. Moreover, the configuration properties are tightly coupled to the application.
The Solution: MicroProfile Config
MicroProfile Config, part of the MicroProfile project, addresses these issues by providing a unified way to handle configuration in Java EE and Jakarta EE applications. It addresses the above issues with a flexible, extensible and standards-based approach.
Here's how you can access configuration using MicroProfile Config:
@Inject
@ConfigProperty(name = "db.url")
private String dbUrl;
Or for programmatic access:
@Inject
private Config config;
public String getDbUrl() {
return config.getValue("db.url", String.class);
}
How MicroProfile Config Works
MicroProfile Config operates on the principle of configuration sources and priorities, offering powerful features. Here’s everything you need to understand the MicroProfile Config API:
- Config Sources: Configuration can come from multiple sources, such as system properties, environment variables, properties files, databases.
- Ordinal: Each config source has a priority, known as ordinal. Higher ordinal values take precedence, overriding those with lower values.
- Converters: Built-in and custom converters allow automatic conversion of configuration values to specific Java types.
- Dynamic Updates: Some config sources can be updated at runtime, with changes automatically reflected in the application.
- Injection: Configuration values can be injected directly into your code using Jakarta CDI.
Why You Should Care
Switching to MicroProfile Config provides a host of benefits that directly address the pain points of traditional configuration management. In particular:
- Simplification: Centralizes configuration management, reducing boilerplate code and potential errors.
- Flexibility: Easily adapt your application for different environments without modifying your code.
- Type Safety: Retrieve configuration values as specific types, helping you catch errors at compile-time rather than runtime.
- Standards-Based: MicroProfile Config is a standard API that is part of MicroProfile, ensuring compatibility across different Java EE and Jakarta EE implementations.
- Dynamic Configuration: Supports runtime updates to configuration, allowing changes to be applied without restarting the application and ultimately improving application flexibility and uptime.
Advanced Features and Considerations
Custom Config Sources
MicroProfile Config allows you to create custom configuration sources. For example, you can source configurations from a database for database-stored configurations:
public class DatabaseConfigSource implements ConfigSource {
@Inject
AmazingDatabaseService dbService;
@Override
public Map<String, String> getProperties() {
return dbService.loadProps();
}
@Override
public String getValue(String propertyName) {
return dbService.getPropertyValue(propertyName);
}
@Override
public String getName() {
return "Database Config Source";
}
}
Custom Converters
You can also implement custom type conversions:
@Priority(100)
public class CustomTypeConverter implements Converter<CustomType> {
@Override
public CustomType convert(String value) {
// Convert string to CustomType
}
Caveats to Consider
While MicroProfile Config offers many advantages, there are a few considerations to keep in mind:
- Learning Curve: While MicroProfile Config simplifies many aspects of configuration management, there is an initial learning curve, especially when first transitioning from traditional configuration management approaches.
- Runtime Dependencies: Make sure your runtime supports MicroProfile Config or include the necessary dependencies. Tip: you can just use Payara Server, Payara Micro or Payara Cloud - they offer excellent choices for this!
- Performance Consideration: While generally negligible, be aware of potential performance impacts when using dynamic configuration sources, especially in high-throughput applications.
- Naming Conventions: Establish clear and consistent naming conventions for your configuration properties to avoid conflicts, improve code readability and maintainability.
- Testing: Ensure test environments are properly configured, including mock configurations for unit testing components that rely on injected configuration values.
Conclusions
MicroProfile Config simplifies configuration management in Java EE and Jakarta EE applications. It provides a unified API that works across different environments, making your applications more adaptable. With MicroProfile Config, you can handle type-safe configurations, making your code safer. You can easily adapt your applications to different deployment scenarios, improving flexibility. MicroProfile Config is a solid choice for your configuration needs, whether you're working on microservices, cloud-native applications or traditional enterprise systems. Start integrating it into your projects today and enjoy smoother, more reliable configuration management.
Remember to use its advanced features and keep security in mind when handling sensitive data. Happy Nugget Friday! Happy Coding!
Related Posts
Nugget Friday - Preventing Memory Leaks with JDBC Connection Pool Management in Payara Server
Published on 15 Nov 2024
by Luqman Saeed
0 Comments
AI Tools for Jakarta EE at the upcoming Virtual Payara Conference
Published on 14 Nov 2024
by Dominika Tasarz
0 Comments
Virtual Payara Conference is coming next month and on Day 2 - Developer Insight - we will help you unlock the future of Jakarta EE development!
AI Tools for Jakarta EE - 12 December 2024, 3:40pm GMT - Register Here!
Join Gaurav Gupta, Senior ...