Nugget Friday - Getting Started with MicroProfile OpenAPI Documentation
Published on 17 Jan 2025
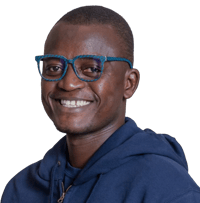
Clear and accurate API documentation is essential. It bridges the gap between your REST APIs and the developers who rely on them, ensuring smooth integration and usage. However, creating and maintaining up-to-date documentation often feels like a daunting task, requiring either tedious manual effort or complex tooling.
In this Nugget Friday, we’ll discuss how you can streamline API documentation to deliver outstanding API experiences with minimal overhead.
The Problem
One of the most important aspects of building REST APIs is having clear, accurate and up-to-date documentation. Without it, your API consumers will struggle to understand and integrate with your services effectively. While frameworks like Jakarta REST (JAX-RS) make it easy to build APIs, documenting them has traditionally required significant manual effort or complex external tools.
The Solution
MicroProfile OpenAPI provides a powerful and flexible way to document your Jakarta REST APIs using a combination of annotations, static files and programmatic models. Let's explore how to get started with the basic documentation mechanisms.
Documenting with Annotations
The simplest way to start is by enhancing your existing Jakarta REST code with OpenAPI annotations. For example:
@Path("/bookings")
@GET
@Operation(
summary = "Get all bookings",
description = "Returns a list of all bookings in the system"
)
@APIResponse(
responseCode = "200",
description = "List of bookings retrieved successfully",
content = @Content(
mediaType = "application/json",
schema = @Schema(implementation = Booking.class)
)
)
public Response getBookings() {
return Response.ok(bookingService.getAllBookings()).build();
}
@Schema(name = "Booking", description = "Represents a booking in the system")
public class Booking {
@Schema(required = true, example = "B123")
private String id;
@Schema(required = true, example = "2024-12-26")
private LocalDate bookingDate;
}
The @Operation annotation provides high-level information about the API endpoint, telling developers what it does (gets all bookings) in both a short summary and longer description. The @APIResponse annotation describes what a successful response looks like - in this case, explaining that a 200 status code means the bookings were retrieved successfully, and specifying that the response will be JSON containing a list of booking objects.
In the data model class, the @Schema annotations provide detailed information about each field, marking required fields and showing example values to help API consumers understand the expected data format. For example, a booking ID might look like "B123", while the booking date follows the ISO-8601 format. This combination of annotations creates clear, structured documentation that helps other developers understand both the API endpoints and the data they'll be working with.
Static Documentation
For more complex scenarios or when you want to maintain documentation separately from code, you can create a static OpenAPI file named openapi.yml (or openapi.yaml/openapi.json) in your META-INF folder:
openapi: 3.1.0
info:
title: Booking API
version: 1.0.0
description: API for managing bookings
paths:
/bookings:
get:
summary: Get all bookings
responses:
'200':
description: List of bookings retrieved successfully
Accessing the Documentation
Once you've documented your API, MicroProfile OpenAPI automatically exposes it at the /openapi endpoint. By default, it serves YAML format, but you can also get JSON by setting the Accept header to application/json.
Configuration Options
MicroProfile OpenAPI provides several configuration options through MicroProfile Config:
# Disable annotation scanning if using only static files
mp.openapi.scan.disable=true
# Specify packages to scan
mp.openapi.scan.packages=com.example.booking,com.example.users
# Add a global server
mp.openapi.servers=https://api.example.com/v1
Why You Should Care
- Zero Additional Runtime Dependencies: MicroProfile OpenAPI integrates with your Jakarta REST application.
- Multiple Documentation Options: Choose between annotations, static files, or a combination of both based on your needs.
- Standards Compliant: Generates OpenAPI 3.1 documentation that works with standard tools like Swagger UI.
- Flexible Configuration: Extensive configuration options let you control exactly what gets documented and how.
Caveats
- Annotation Processing: If you disable annotation scanning, make sure your static documentation is complete.
- Version Compatibility: Be sure your tooling supports OpenAPI 3.1 format.
- Performance Impact: While minimal, annotation scanning can add a small overhead during startup.
Conclusions
MicroProfile OpenAPI makes it easy to maintain high-quality API documentation alongside your code. Whether you prefer annotation-driven documentation, static files, or a mix of both, you can build a documentation strategy that works for your team and your consumers. Download your copy of Payara Platform Community application server and start documenting your APIs today. Happy coding!
Related Posts
A More Flexible Way to Deploy Jakarta EE Apps: Introducing Pay As You Go Pricing for Payara Cloud
Published on 05 Dec 2024
by Luqman Saeed
0 Comments
Getting Started with Observability in Jakarta EE Applications: Why Observability Matters
Published on 22 Nov 2024
by Luqman Saeed
0 Comments