MicroProfile OpenAPI in the Payara Platform
Originally published on 28 Jun 2018
Last updated on 13 Jul 2018
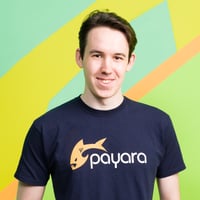
One of the new features in Payara Platform version 5.182 is full compatibility with MicroProfile 1.3. In this blog post, I will introduce OpenAPI 1.0, a new API in MicroProfile 1.3. This functionality is available in both Payara Server and Payara Micro in version 5.182. It's also available in version 4.1.2.182 for Payara Support customers.
What is OpenAPI?
The OpenAPI specification itself is unrelated to MicroProfile. It's a specification designed to provide a standard format for documenting REST API services. An OpenAPI document looks similar to the following:
openapi: "3.0.0" info: version: 1.0.0 title: Swagger Document servers: - url: http://test.payara.fish/v1 paths: /hello: get: summary: "Returns a hello world message." operationId: helloWorld parameters: - name: count in: query description: "How many instances of the message to return." required: false schema: type: integer responses: 200: description: "A hello world message." content: text/plain: schema: type: string 500: description: "An unexpected error." content: application/json: schema: $ref: "#/components/schemas/Error" components: schemas: Error: required: - code - message properties: code: type: integer message: type: string
This document contains things such as:
- a list of common objects
- a list of servers the API is available from
- a list of each path in the API as well as what parameters it accepts
This document is extremely useful since, as it follows a standard, it can be used in a range of tools such as those provided by the Swagger suite. These let you do all sorts of things such as design, edit and test a REST API documented by an OpenAPI document.
How Does This Relate to MicroProfile?
MicroProfile designed the MicroProfile OpenAPI specification to extend the general OpenAPI specification and integrate it into MicroProfile applications. The specification states that any implementing servers should host an endpoint at /openapi
which will provide an OpenAPI document built from a deployed application. When you deploy an application, this document will be built automatically according to the application contents. The document can then be edited or used however you like. The specification also illustrates a few methods of editing this document. You could for example add a description to an endpoint, or a new title for the document so that the document hosted at the /openapi
endpoint appears in any way you like.
How Can I Use MicroProfile OpenAPI in the Payara Platform?
No configuration is needed for the OpenAPI implementation in the Payara Platform, you need just deploy an application! The OpenAPI is included in Payara Micro and Payara Server, so which one you use doesn't matter. For a full example of the usage, see the specification documentation.
Let's say you deployed an application with only the following classes:
import javax.ws.rs.ApplicationPath; import javax.ws.rs.core.Application; @ApplicationPath("/api") public class TestApplication extends Application { }
import javax.ws.rs.Path; import javax.ws.rs.GET; @Path("/hello") public class TracedResource { @GET public String helloWorld() { return "Hello World!"; } }
With this application deployed, visiting http://localhost:8080/openapi/ will produce the following document:
openapi: 3.0.0 info: title: Deployed Resources version: 1.0.0 servers: - url: http://localhost:8080/app description: Default Server. paths: /api/hello: get: operationId: helloWorld responses: default: description: Default Response. content: '*/*': schema: type: string components: {}
This is useful, but not as useful as it could be. Often you'll want descriptions, and more in depth descriptors for the objects in the application (more similar to the first document in this blog). There are various ways of doing this. Each of the following configuration sources are applied in order:
- Configuration properties provided via the MicroProfile Config API. This allows you to do lots from disabling application scanning to including extra servers in the document.
- An
OASModelReader
implementation in the application. This provides a base document to be built upon for the final output. - A static OpenAPI document provided in the application. This does the same as the former, but in a slightly different format.
- Annotations on the endpoint methods or object classes. This is the most idiomatic way of editing the document with minor changes.
- An
OASFilter
implementation in the application. This will visit each element in the document and either remove it or edit it programmatically.
A brief example of each can be seen below. For a more detailed example utilising each of these, see the following example: https://github.com/payara/Payara-Examples/tree/master/microprofile/openapi-example.
Config Properties
The MicroProfile OpenAPI specification includes a few properties for the MicroProfile Config API. These can be included from any of the sources defined in the MicroProfile Config API. For example, in a config file stored in src/main/resources/META-INF/microprofile-config.properties
.
An example configuration property might look as follows:
# Whether to disable the scanning of application classes mp.openapi.scan.disable=false
OASModelReader and OASFilter Implementations
MicroProfile OpenAPI provides two interfaces for the application developer to implement:
org.eclipse.microprofile.openapi.OASFilter
org.eclipse.microprofile.openapi.OASModelReader
Each of these have a similar function, but are applied at different times. An implementation of OASModelReader
will create an initial OpenAPI document to build the final document from. An implementation of OASFilter
will be used to visit every object in the OpenAPI document before it is published. This allows final configuration changes to be made.
When implementing each of these, the relevant interface should be implemented, and a config property should be specified in the Config API (often in microprofile-config.properties
):
# Configure the processing classed defined to edit the OpenAPI document. mp.openapi.model.reader=fish.payara.examples.microprofile.openapi.ModelReader mp.openapi.filter=fish.payara.examples.microprofile.openapi.Filter
A Static OpenAPI Document
A static OpenAPI document file can be placed in src/main/resources/META-INF
and called any of openapi.yaml
, openapi.yml
or openapi.json
(and represented in the appropriate format of YAML or JSON). When this happens, this document will be used before any application processing. This means that if you want, you can take the document produced by the application initially, then remove any OpenAPI annotations present, edit the document as you wish and place it in the resources folder again to produce the same output!
Annotations
MicroProfile OpenAPI defines several annotations that can be used to augment the produced OpenAPI document. These are detailed in the MicroProfile OpenAPI specification, but an example of them is as follows:
import javax.ws.rs.GET; import javax.ws.rs.Path; import org.eclipse.microprofile.openapi.annotations.Operation; @Path("/hello") public class TestResource { @GET @Operation(operationId = "hello world") public String helloWorld() { return "Hello World!"; } }
This helps make small tweaks to the produced document, and in the most non-intrusive way of editing the output.
The OpenAPI service in Payara Platform
The OpenAPI service is enabled by default in Payara Server and Payara Micro. The service can be controlled by two new asadmin commands. These need very little explanation, so here they are for reference!
set-openapi-configuration --enabled=true/false
get-openapi-configuration
When the OpenAPI service is disabled, no applications deployed during this period will have OpenAPI documents produced, and the endpoint will return a 403 response. A quirk of the service is that when it is enabled again, any applications deployed before it was disabled will still have their document shown, whereas any deployed while it was disabled will need redeploying. Outside of this functionality, the most recently deployed application will have it's OpenAPI document shown.
A Laid-Back Approach to REST Documentation
The new MicroProfile Open API service in Payara Platform enables building and serving OpenAPI documents out of the box. Before the OpenAPI implementation, documents would have to be produced manually, or using an external tool and hosted as part of the application. This new integration eases the production of the document, and makes it feel more native to a MicroProfile application.
Related Posts
Nugget Friday - Getting Started with MicroProfile OpenAPI Documentation
Published on 17 Jan 2025
by Luqman Saeed
0 Comments
Web Server vs. Application Server: What's the Difference?
Published on 16 Jan 2025
by Chiara Civardi
0 Comments
Planning to develop and deploy an application but unsure where to start? Whether you’re new to software engineering or managing a team of developers for the first time, you’ve likely heard you need a server—but what kind? Aren’t all servers ...