Using Payara Embedded as an Arquillian Container Inside IntelliJ IDEA
Published on 22 Sep 2016
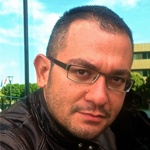
With this article, I'm going to integrate Payara Embedded with Arquillian by having it defined inside a sample Maven based application - source code available here - which employs an integration test implemented by the Arquillian framework. You can also find our previous post about Arquillian and the Payara Server available here, but this time I’ll take it one step further and move onto the IDE side. In this example, I will execute tests just like any JUnit test; meaning the test will be executed directly through the IDE with help of a right-click. I will also configure Payara Embedded as the Arquillian container inside the IDE.
Arquillian is an integration and functional testing framework that can be used for testing Java middleware. You can find more information on what it can be used for on their website here. Intellij IDEA is one of the most powerful IDEs on the market and, with its 2016.2 release, it now offers enhanced support for configuring Arquillian containers.
Setting up Project Structure and Dependencies
My sample project is a web application architected with Maven and it contains a simple domain object, its DAO implementation, its integration test, and the Arquillian configuration.
Let’s first start detailing the Maven dependencies. It’s easy to integrate Arquillian via Maven by adding its universal BOM dependency management definition as given follows:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.jboss.arquillian</groupId>
<artifactId>arquillian-bom</artifactId>
<version>1.1.11.Final</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Throughout this dependency management section, I will use the arquillian-junit-container
dependency by adding it to my project. It provides the integration with JUnit for implementing our tests. It also transitively depends on Arquillian core and ShrinkWrap APIs so I don’t need to add those as separate dependencies.
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<scope>test</scope>
</dependency>
The other dependencies that I’m going to add separately are: arquillian-persistence-dbunit
, which is a part of the Arquillian persistence extension project that helps with implementing the tests where a persistence layer is involved and arquillian-glassfish-embedded-3.1
dependency, which provides container integration for unit tests and, in our example, it will provide the support for integrating of Payara Embedded into our tests.
<dependency>
<groupId>org.jboss.arquillian.extension</groupId>
<artifactId>arquillian-persistence-dbunit</artifactId>
<version>1.0.0.Alpha7</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.container</groupId>
<artifactId>arquillian-glassfish-embedded-3.1</artifactId>
<version>1.0.0.CR4</version>
<scope>test</scope>
</dependency>
Since I’ll be using Payara Embedded as a container for running Arquillian tests, I’m also adding it as a dependency with test scope.
<dependency>
<groupId>fish.payara.extras</groupId>
<artifactId>payara-embedded-all</artifactId>
<version> 4.1.1.163.0.1</version>
<scope>test</scope>
</dependency>
The rest of the dependencies that we have with test scope are JUnit and H2 dependencies. H2 will be used as in-memory database to have the Arquillian test running on. Stay tuned for the connection pool configuration to see the DB configuration in action.
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.191</version>
<scope>test</scope>
</dependency>
For integrating Arquillian tests into the Maven’s build process, we also need to define the maven-surefire-plugin
with the qualifier configuration for Arquillian as follows.
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<systemProperties>
<arquillian.launch>glassfish-embedded</arquillian.launch>
</systemProperties>
</configuration>
</plugin>
The keyword glassfish-embedded
will be defined in Arquillian configuration file, which I’ll be mentioning in a bit.
You can see the complete pom.xml here.
Setting up Arquillian Configuration
Arquillian can be easily configured via an XML file and a sample configuration for my project is given below. Within the container configuration, I’m providing a GlassFish resource configuration file via a path definition. This GlassFish configuration will contain a JDBC resource and a connection pool definition that will be used by our persistence configuration.
<arquillian xmlns="http://jboss.org/schema/arquillian"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://jboss.org/schema/arquillian
http://jboss.org/schema/arquillian/arquillian_1_0.xsd">
<defaultProtocol type="Servlet 3.0"/>
<container qualifier="glassfish-embedded">
<configuration>
<property name="bindHttpPort">7070</property>
<property name="resourcesXml">
src/test/resources/glassfish-resources.xml
</property>
</configuration>
</container>
</arquillian>
A sample GlassFish configuration file is shown below. A JDBC resource is created with JNDI name jdbc/payaraEmbedded
is created and it’s backed up with the in-memory H2 database configuration.
<!DOCTYPE resources PUBLIC
"-//GlassFish.org//DTD GlassFish Application Server 3.1
Resource Definitions//EN"
"http://glassfish.org/dtds/glassfish-resources_1_5.dtd">
<resources>
<jdbc-resource pool-name="ArquillianEmbeddedH2Pool"
jndi-name="jdbc/payaraEmbedded"/>
<jdbc-connection-pool name="ArquillianEmbeddedH2Pool"
res-type="javax.sql.DataSource"
datasource-classname="org.h2.jdbcx.JdbcDataSource">
<property name="user" value="sa"/>
<property name="password" value=""/>
<property name="url" value="jdbc:h2:mem:payaraEmbedded"/>
</jdbc-connection-pool>
</resources>
Domain model and DAO implementation
To demonstrate the features of Arquillian, I’m going to create a simple domain class named Person
, where it contains the properties name and lastName
@Entity
@NamedQueries(
@NamedQuery(name = "Person.getAll",
query = "select p from Person p"))
public class Person {
@Id
@GeneratedValue
private long id;
private String name;
private String lastName;
…
}
The DAO implementation for the Person
is also a simple one that just contains the getAll()
method for retrieving all persisted data.
@Stateless
public class PersonDao {
@PersistenceContext
private EntityManager entityManager;
public List<Person> getAll() {
return entityManager.createNamedQuery("Person.getAll",
Person.class).getResultList();
}
}
Testing the DAO
There is not much to test inside the DAO since it only offers getAll() method but we should always test what we have at hand. To test fetching the all persisted data, an Arquillian test is implemented as follows:
@RunWith(Arquillian.class)
public class PersonDaoTest {
@EJB
private PersonDao personDao;
@Deployment
public static WebArchive createDeployment() {
return ShrinkWrap.create(WebArchive.class, "arquillian-example.war")
.addClass(Person.class)
.addClass(PersonDao.class)
.addAsResource("test-persistence.xml",
"META-INF/persistence.xml");
}
@Test
@UsingDataSet("datasets/person.yml")
public void shouldReturnAllPerson() throws Exception {
List<Person> personList = personDao.getAll();
assertNotNull(personList);
assertThat(personList.size(), is(1));
assertThat(personList.get(0).getName(), is("John"));
assertThat(personList.get(0).getLastName(), is("Malkovich"));
}
}
The createDeployment()
method creates a micro-deployment that bundles the Person
and PersonDao
classes and respective persistence configuration file together.
The @UsingDataSet
annotation is DBUnit specific which loads the data defined in file person.yml
into the database before the test execution. With this approach after invoking the DAO’s getAll()
method, I can expect that the list returned not to be null
and containing the Person object that I defined with the yaml file. The content of the person.yml
is given below. It could also be defined in different formats, such as JSON or XML.
Person:
- id: 1
name: "John"
lastname: "Malkovich"
Running the test via Maven
Since I integrated maven-surefire-plugin
with Arquillian configuration, running tests is simple as invoking the test phase of Maven lifecycle.
Running the test inside IDE
Running the PersonDaoTest inside the IDE is simple as a right-click as seen in the figure below:
But of course some configuration is needed in order to proceed. By clicking Run ‘PersonDaoTest’ from the menu given above, you will come across with the UI in the following screenshot, stating that Arquillian container configuration should be setup first in order to continue.
After clicking + on top left, select Manual container configuration from the menu.
In the dependencies section, click + to add Payara Embedded maven dependency.
Inside the popup, just type fish.payara.extras:payara-embedded-all:4.1.1.163.0.1 into the combo box and select OK.
After getting dependency defined, specify Arquillian.xml container qualifier as glassfish-embedded
, which maps to our qualifier configuration defined in the arquillian.xml:
Conclusion
So after getting everything configured, there is only one thing left, which is running the test with just a right-click. With the execution of the test, we will have our JDBC resource and connection pool defined; will have our Person table created in our in-memory H2 database; will have our sample person inserted with the help of @UsingDataSet, and will have that single person which we defined in the yaml file fetched from the DB through our DAO layer.