How to prevent runtime type erasure using GenericEntity in Jakarta REST in Jakarta EE 10
Published on 09 Mar 2023
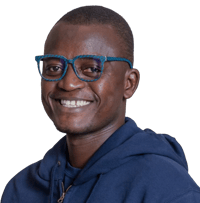
Java generics is a great feature that allows you to have compile time checks for generics. However, due to historical reasons of backward compatibility, type information for generics is erased at runtime. A lot of the time this shouldn’t be of much concern. But there are a few cases where type information is needed at runtime for some kind of decision.
One such situation is in Jakarta REST when the jakarta.ws.rs.core.Response object is used to return a generic collection of a specific type. For example the code below shows the creation and return of a Response object that has a list of HelloEntity as the return payload to the client.
List<HelloEntity> helloEntities = greetingService.loadSampleEntities();
return Response.ok(helloEntities).build();
The problem with the above is that type erasure removes the type from the list such that at runtime the passed list becomes List<?> instead of the specific Java type HelloEntity passed at compile time. For a lot of cases this may not be of concern. But if you have a complex or very custom case where the generic information is needed at runtime to fetch the exact jakarta.ws.rs.ext.MessageBodyWriter, then this could be a very big problem.
To get around this problem, the Jakarta API has the jakarta.ws.rs.core.GenericEntity<T> wrapper for wrapping generic types, as shown in the code snippet below.
List<HelloEntity> helloEntities = greetingService.loadSampleEntities();
GenericEntity<List<HelloEntity>> entity = new GenericEntity<>(helloEntities) {};
return Response.ok(entity).build();
The original HelloEntity list is wrapped in a GenericEntity created as an anonymous class. This new object is then passed as the entity of the returned Response object. With this construct, the typed information of the original helloEntities list is not lost and can be retrieved at runtime. So next time you need to maintain generic information at runtime in Jakarta REST, give GenericEntity a look.
Found this useful? Try more of ourJakarta EEcontent:
Related Posts
Join our webinar! Modernizing Enterprise Java Applications: Jakarta EE, Spring Boot, and AI Integration
Published on 12 Feb 2025
by Nastasija Trajanova
0 Comments
Join us for a power-packed webinar in collaboration with DZone, where we’ll dive into the latest innovations in Jakarta EE, Spring Boot, and AI integration. Get ready for live coding, real-world case studies, and hands-on insights into the ...
10 Ways Jakarta EE 11 Modernizes Enterprise Java Development
Published on 10 Feb 2025
by Luqman Saeed
0 Comments