Payara for Beginners - a Simple JBatch Schedule
Originally published on 05 Jan 2016
Last updated on 20 Apr 2017
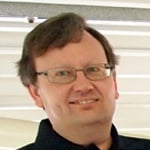
Firstly, we need to create a java class that appends the current datetime to a file.
JBatch implement two variations of Job step: Chunks - which are built around the traditional batch process of reader, processor and writer elements – and Batchlets for simple tasks.
For the simple process appending a datetime to a file we will be using a Batchlet.To correctly implement a Batchlet we must define process() and stop() methods.
@Dependent
@Named("TestBatchlet")
public class TestBatchlet implements Batchlet {
@Inject
private JobContext jobCtx;
@Override
public String process() throws Exception {
String filename = jobCtx.getProperties().getProperty("outfile");
String timestamp = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date());
BufferedWriter output = new BufferedWriter(new FileWriter(filename, true));
output.append(timestamp);
output.newLine();
output.close();
return "COMPLETED";
}
@Override
public void stop() throws Exception {
}
}
Next, we need to define the steps that make-up our batch job using the Job Specification Language (JSL). The below JSL defines our single-step job and also the value of the outfile property used by the Batchlet.The JSL must be held in an XML file located in the META-INF/batch-jobs directory.
BatchTest.xml
<?xml version="1.0" encoding="UTF-8"?>
<job id="testjob" xmlns="http://xmlns.jcp.org/xml/ns/javaee" version="1.0">
<properties>
<property name="outfile" value="\tmp\output.txt"/>
</properties>
<step id="teststep">
<batchlet ref="TestBatchlet"/>
</step>
</job>
Finally, we will create an EJB to trigger our job every 30 seconds
@Stateless
public class TestSchedule {
@Schedule(second = "*/30", minute = "*", hour = "*") // Thirty second intervals
public void processFiles() {
JobOperator jobOperator = BatchRuntime.getJobOperator();
jobOperator.start("BatchTest", null);
}
}
Deploying the above will append the current datetime to the file specified in the XML at 30 second intervals:
2015-12-21 15:06:00
2015-12-21 15:06:30
2015-12-21 15:07:00
2015-12-21 15:07:30
2015-12-21 15:08:00
2015-12-21 15:08:30
And this is all that is required to implement the most basic batch process!
Want to learn more about JBatch? Check out Batch Applications in Java EE 7 - Undertanding JSR 352 Concepts article by Arun Gupta on the GlassFish blog; or watch the 'Batch Applications for the Java Platform 1.0' video by Chris Vignola.
Related Posts
Moving Beyond GlassFish - Here's Why and How
Published on 11 Nov 2024
by Chiara Civardi
0 Comments
If you’re still managing Java applications on GlassFish middleware, you might be hitting some roadblocks, such as difficulties with automation, lack of integrated monitoring and official support and limited abilities with modern tools. However, ...
The Payara Monthly Catch - October 2024
Published on 30 Oct 2024
by Chiara Civardi
0 Comments