Nugget Friday - Statements Before super()
Published on 26 Jul 2024
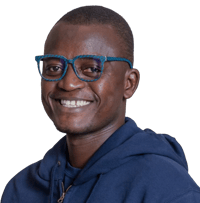
As Java developers, we’ve long grappled with the constraints of constructor logic and initialization. In the past, if you needed to perform any operations before invoking the superclass constructor (via super()) in any class, you were often forced to rely on helper methods or extra constructors. This mostly ended up with cluttered, hard to read code as you needed to duplicate constructors or find workarounds. Let's look at how to address this in today's Nugget Friday!
The Problem
When developing Java classes that extend other classes, there are often constraints on the values passed to constructors. In particular, the restriction of having the super()
call as the first statement in a constructor means that developers have to rely on cumbersome workarounds, resulting in cluttered and less readable code. Let's look at this example.
In the following class, we need to check the passed value to the constructor is positive. Such a requirement could be expressed as follows:
public class PositiveBigInteger extends BigInteger {
public PositiveBigInteger(long value) {
super(String.valueOf(validatePositive(value)));
}
private static long validatePositive(long value) {
if (value <= 0) {
throw new IllegalArgumentException("Value must be positive");
}
return value;
}
}
As you can see, we needed to rely on a static method for the validation and the super()
call had to be the first statement in the constructor. While this approach works, it's unnecessarily noisy and reduces readability, especially for such a trivial requirement.
The Solution
JEP 447, offered in Java 22, introduces the statements before super() in a constructor. This allows us to carry out any custom logic needed before invoking the superclass constructor. This feature simplifies the initialization logic and makes the code cleaner and more readable. With this new feature, we can rewrite the above code as follows:
public class PositiveBigInteger extends BigInteger {
public PositiveBigInteger(long value) {
if (value <= 0)
throw new IllegalArgumentException("non-positive value");
super(String.valueOf(value));
}
}
How neat, simple and readable is that? The caveat with this feature is that your statements before the super() invocation should not try to access instance variables of the class. This restriction ensures that the object is not partially constructed when these statements are executed.
Thanks to JEP 447, you can write clearer constructor code that requires less cognitive overhead to read and reduces boilerplate. As a result, code is easier to read and to maintain.
That is it for today’s Nugget. See you next Friday. Happy Coding!
Related Posts
10 Ways Jakarta EE 11 Modernizes Enterprise Java Development
Published on 10 Feb 2025
by Luqman Saeed
0 Comments
10 Reasons Why Java is the Future of Enterprise App Development in 2025
Published on 03 Feb 2025
by Luqman Saeed
0 Comments