Nugget Friday: Simplify Module Imports in Java 23
Published on 23 Aug 2024
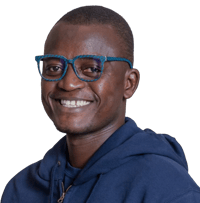
Welcome to this week’s Friday Nugget! In today’s post, we’re focusing on a new feature coming in Java 23 that’s set to make your coding life a little easier, especially if you’ve ever found yourself frustrated by the clutter of import statements: module import declarations. By leveraging such tool, you can streamline your Java code, reduce verbosity and enhance readability, making your development process more efficient.
The Problem
Java has always been a bit verbose when it comes to importing classes and interfaces. While experienced developers may have grown accustomed to managing numerous import statements at the top of their files, this can be daunting for beginners. Even the wildcard import option (import java.util.*
) has its limitations, often leading to cluttered code when diverse parts of a module's API are used, with multiple import statements at the top of your files. This not only hampers readability but also introduces cognitive noise, making it harder to focus on the logic of the code itself. Moreover, it can be particularly frustrating for beginners who are just starting to explore the Java ecosystem.
Take the following example:
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
String[] fruits = new String[] { "apple", "berry", "citrus" };
Map<String, String> m =
Stream.of(fruits)
.collect(Collectors.toMap(s -> s.toUpperCase().substring(0,1),
Function.identity()));
Even though the code is relatively short, the multiple import statements add unnecessary bulk. While import java.util.*
could simplify this slightly, it would also pull in everything from the java.util
package, much of which might never be used, further cluttering the namespace. This presents a clear challenge: how can we strike a balance between too many specific imports and overly broad wildcard imports? What’s the middle ground?
The Solution
The upcoming Java 23, expected for release in September 2024, introduces a preview language feature called module import declarations (JEP-476) to address this issue. This feature lets you import all the public top-level classes and interfaces from an entire module at once using a single, concise statement.
How Module Import Declarations Work
The new syntax for module import declarations is straightforward:
import module module_name;
This statement will import all the public types from:
- The packages directly exported by the specified module.
- The packages exported by modules that are transitively required by the specified module.
This means you can access a wide range of classes and interfaces without the need for multiple import statements, making your code cleaner and easier to read.
Check this example on how you can use the new module import declaration:
import module java.base; // Imports all public types from java.base and its transitive dependencies
// Now you can use List, Map, Stream, Path, etc., without additional import statements
List<String> myList = new ArrayList<>();
Benefits of Module Import Declarations
- Simplified Code: Reduces the number of import statements, leading to cleaner and more readable code.
- Easier Learning Curve: Helps beginners use third-party libraries and fundamental Java classes without getting bogged down by package hierarchies.
- Improved Prototyping and Exploration: Simplifies the process of experimenting with new APIs and language features.
Important Considerations
While module import declarations offer significant benefits, there are a few important considerations to keep in mind:
- Ambiguous Imports: Importing multiple modules could lead to name conflicts if different packages contain types with the same simple name. In such cases, you'll need to use single-type-import declarations to resolve the ambiguity.
- Preview Feature: Module import declarations are a preview feature in Java 23 and are disabled by default. You'll need to enable preview features when compiling and running your code to use them.
Conclusions
The introduction of module import declarations in Java 23 marks a significant step towards simplifying Java development, especially for those new to the language. Module import declarations will offer a convenient way to work with modules and their APIs, promoting cleaner code and a smoother professional development experience, easing the learning curve. While it's still a preview feature, it shows promising potential for simplifying Java development in the future, leading to a more efficient and accessible programming experience.
Happy Coding!
Related Posts
Nugget Friday - Exploring Jakarta RESTful (JAX-RS) Web Services Validation
Published on 13 Sep 2024
by Luqman Saeed
0 Comments
Mastering Java Frameworks: Power Up Your Jakarta EE Skills As A Spring Boot Developer
Published on 10 Sep 2024
by Chiara Civardi
0 Comments