Nugget Friday - Jakarta JSON Binding: Simplifying JSON Serialization and Deserialization
Published on 11 Oct 2024
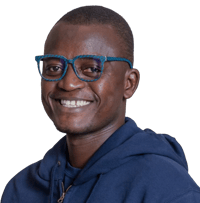
In today's Nugget Friday, we're tackling a common pain point that nearly every Java developer has faced before: the tedious task of converting Java objects to JSON and back. If you are tired of writing glue code to convert your Java objects to and from JSON, then stick around for this nugget.
The Problem
JSON (JavaScript Object Notation) has become the de facto standard for data exchange in modern web applications. However, converting Java objects to JSON and back can be a tedious and error-prone process. We often find ourselves writing boilerplate code to handle this conversion, leading to increased development time and potential bugs.
The Solution: Jakarta JSON Binding
Jakarta JSON Binding provides a standardized, annotation-based approach to JSON serialization and deserialization in Java applications. It offers a simple yet flexible API that can handle most common use cases out of the box, while also providing customization options for more complex scenarios.
How It Works
Let's look at a simple example of how JSON-B works:
private String name;
private int age;
// Obligatory Getters and setters...
}
// Serialization
Jsonb jsonb = JsonbBuilder.create();
Person person = new Person("John Doe", 30);
String json = jsonb.toJson(person);
// Deserialization
Person deserializedPerson = jsonb.fromJson(json, Person.class);
In this example, JSON-B automatically handles the conversion between Java objects and JSON strings without any additional configuration.
Key Features
-
Default Mapping: JSON-B provides sensible defaults for mapping Java objects to JSON. It handles common Java types, collections, arrays, and even nested objects out of the box.
-
Customization: When you need more control, JSON-B offers various annotations and configuration options:
-
@JsonbProperty: Customize property names
-
@JsonbTransient: Exclude properties from serialization/deserialization
-
@JsonbDateFormat: Specify custom date formats
-
@JsonbNillable: Control null value handling
-
-
Adapters and Serializers: For complex types or third-party classes, you can use custom adapters or serializers to define exactly how the conversion should happen.
-
Integration with JSON Processing: JSON-B works with Jakarta JSON Processing (JSON-P), allowing you to work with both APIs in the same application.
Why You Should Care
-
Simplicity: JSON-B significantly reduces the amount of code you need to write for JSON handling.
-
Standardization: As part of the Jakarta EE platform, JSON-B provides a standard API that works across different implementations.
-
Flexibility: While it offers great defaults, JSON-B also provides extensive customization options to handle complex scenarios.
-
Performance: JSON-B implementations are optimized for performance, handling large amounts of data efficiently.
Advanced Features
Polymorphic Type Handling
JSON-B supports polymorphic types through the @JsonbTypeInfo and @JsonbSubtype annotations. This allows you to correctly serialize and deserialize objects in an inheritance hierarchy:
@JsonbSubtype(alias = "car", type = Car.class),
@JsonbSubtype(alias = "bike", type = Bike.class)
})
public abstract class Vehicle { ... }
public class Car extends Vehicle { ... }
public class Bike extends Vehicle { ... }
Custom Instantiation
For classes without a no-arg constructor, you can use the @JsonbCreator annotation to specify a custom constructor or factory method:
private final String name;
private final int age;
@JsonbCreator
public ImmutablePerson(@JsonbProperty("name") String name,
@JsonbProperty("age") int age) {
this.name = name;
this.age = age;
}
}
Caveats
-
Learning Curve: While JSON-B is designed to be intuitive, there's still a learning curve, especially when dealing with complex customizations.
-
Performance Overhead: For extremely performance-critical applications, the convenience of JSON-B might come with a slight overhead compared to hand-optimized JSON parsing.
-
Version Compatibility: Make sure your JSON-B implementation version is compatible with your Jakarta EE version to avoid unexpected issues.
Conclusion
Jakarta JSON Binding simplifies JSON handling in Java applications, offering a powerful yet easy-to-use API for serialization and deserialization. Whether you're working on a small project or a large enterprise application, JSON-B can significantly reduce the complexity of your JSON-related code.
Using this API, you can focus more on your business logic and less on the gymnastics of JSON conversion. It's a valuable tool in any Java developer's toolkit, especially when working with RESTful web services or any JSON-based data exchange.
So, next time you find yourself writing custom JSON parsing code, remember that Jakarta JSON Binding might just be the solution you need!
Happy coding, and see you next Friday for another nugget.
Related Posts
Accelerate Application Development with AI
Published on 16 Jan 2025
by Gaurav Gupta
0 Comments
Join our webinar! Zero Ops, Maximum Impact: Build GenAI RAG Apps with Jakarta EE
Published on 13 Jan 2025
by Dominika Tasarz
0 Comments
Want to build powerful AI applications that can intelligently search and analyze your internal documents?
Join our online event on Thursday the 23rd of January (REGISTER HERE) to learn how to create a serverless Retrieval Augmented Generation ...