Nugget Friday - InputStream To String Post Java 9
Published on 12 Jul 2024
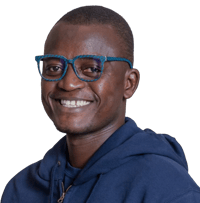
It's Nugget Friday again, and we're excited to bring you another valuable titbit to our beloved coders! In this instalment of our Friday Nugget series, we're diving into a common yet essential task for developers, especially when handling data read from a file, network or database: converting an InputStream to a String in Java. Knowing how to do this is crucial for efficiently managing IO and data processing tasks.
The Problem
One of the criticisms of Java has been its tendency to get quite verbose for certain tasks. One of them can be found precisely in the realm of IO. Nearly anything to do with reading and writing binary data was a bit of royal pain. You needed to contend with the not so trivial ceremonial code. As an example, ignore the new HTTP client introduced in Java 11 for a moment (spoiler alert: this will come in a future nugget) and let's say we are making a call to the Dummy JSON recipe API using the venerable URI class as follows.
InputStream inputStream = new URI("https://dummyjson.com/recipes/search?q=chicken").toURL().openStream();
We have an InputStream that is a binary representation of the data from the API. To convert this to String pre Java 9, you would be doing the following:
try (InputStream inputStream = new URI("https://dummyjson.com/recipes/search?q=chicken").toURL().openStream()) {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
StringBuilder builder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
builder.append(line);
}
String text = builder.toString();
} catch (Exception e) {}
} catch (Exception ex) {}
That is a mouthful, I must admit. You had to contend with two *Reader classes and then a StringBuildder to append the lines from the BufferedReader to. Needless to say, the above code could be made simpler.
The Solution
Java 9 added the readAllbytes method that returns a byte[] to the InputStream class. The String class has a constructor for instantiating a new String object that takes a byte array.
Pairing these two constructs, we can refactor the above code block to the following:
try (InputStream inputStream = new URI("https://dummyjson.com/recipes/search?q=chicken").toURL().openStream()) {
String response = new String(inputStream.readAllBytes());
System.out.println(response);
} catch (Exception ex) {}
Now that is a breath of fresh air. Easy to read and comprehend without much cognitive overhead.
So, there you have it. Converting an InputStream to a String has been reduced from needing all the ceremonial *Readers and StringBuilder to just a line. How’s that for readability and conciseness!
That’s it for today. Stay tuned as we break down the steps and share some tips to make your coding life easier with our Nugget Friday. And if you missed last week's episode, check it out here. Happy Coding!
Related Posts
You don’t need to be in the money to be in the cloud
Published on 28 Aug 2024
by Chiara Civardi
0 Comments
Nugget Friday: Simplify Module Imports in Java 23
Published on 23 Aug 2024
by Luqman Saeed
0 Comments