Consuming a REST Service
Originally published on 07 Sep 2018
Last updated on 18 May 2020
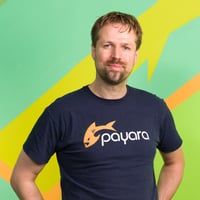
A REST Service in Java EE can be created using JAX-RS. The contents of such service can be consumed using ordinary HTTP requests to a URL. URLs are typically kept simple and have a logical pattern, so it's easy to type them manually in e.g. a browser. This is different from SOAP, which essentially uses HTTP as well, but is designed to be rather complex and therefor making it not so easy to quickly test something in a browser.
What Ways are There to Request a URL?
There is a very large number of tools that allow us to request a URL and see its response. Among the more popular ones are:
- Typing the URL in a browser (e.g. Chrome, FireFox, Safari)
- The wget command line tool
- The curl command line tool
- The Java JAX-RS client builder
- The Java type-safe MicroProfile Rest Client
- The Java HtmlUnit library
Example
For our first example we'll be demonstrating how to consume a REST service via a browser.
We'll start with defining the REST Service (JAX-RS resource) itself as follows:
@Path("/resource") @Produces(TEXT_PLAIN) public class Resource { @GET @Path("hi") public String hi() { return "hi!"; } }
Next we'll create an activator class that activates JAX-RS itself:
@ApplicationPath("/rest") public class JaxRsActivator extends Application { }
When we bundle this together into a war called "simple-get" and deploy it to a default Payara server instance, we can request http://localhost:8080/simple-get/rest/resource/hi and the browser will respond with rendering "hi!":
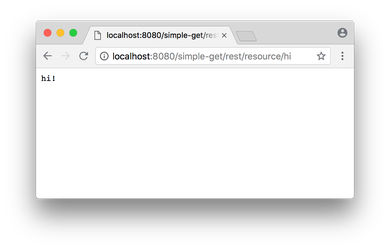
When using the command line, we can use the wget command to achieve consume the REST Service. This can be done as follows:
wget -S -O - http://localhost:8080/simple-get/rest/resource/hi
This will print something like the following to stdout:
HTTP/1.1 200 OK Server: Payara Server 5 #badassfish X-Powered-By: Servlet/4.0 JSP/2.3 (Payara Server 5 #badassfish Java/Oracle Corporation/1.8) Content-Type: text/plain Content-Length: 3 X-Frame-Options: SAMEORIGIN hi!
For the next example we'll be looking at how to consume a rest service programmatically via Java.
From a standalone Java SE application, but also from a Java EE application, this can be done using the JAX-RS Client Builder API as follows:
String response = ClientBuilder.newClient() .target( URI.create(new URL("http://localhost:8080/simple-get/rest/resource/hi").toExternalForm())) .request(TEXT_PLAIN) .get(String.class);
After executing this code the string "response" will be set to the "hi!" that was returned from our service.
The full source of this application can be found on GitHub, along with an automated tests that demonstrates how the application is supposed to be called:
https://github.com/javaee-samples/javaee7-samples/tree/master/jaxrs/simple-get
Related Posts
The Payara Monthly Catch - November 2024
Published on 28 Nov 2024
by Chiara Civardi
0 Comments
A Preview of Jakarta REST (JAX-RS) 4.0 in Jakarta EE 11
Published on 13 Nov 2024
by Luqman Saeed
0 Comments
The latest version of Jakarta REST (formerly Java API for RESTful Web Services, aka JAX-RS), Jakarta REST 4.0, will bring some notable improvements and changes as part of Jakarta EE 11. This release focuses on modernizing the specification by ...