Nugget Friday - Simplifying Multiline Strings with Java Text Blocks
Published on 20 Sep 2024
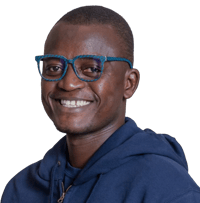
Working with multiline strings in Java has historically been a challenge, often resulting in messy code that is hard to read and maintain. Whether you're dealing with SQL queries, JSON or HTML templates, managing escape characters and manual line breaks is a cumbersome process. In today's Nugget Friday, we'll look at how Text Blocks can redefine how developers handle multiline strings, making code cleaner, more readable and easier to maintain.
The Problem
Handling multiline strings in Java has traditionally been a pain point for us developers. For example, consider this JSON string:
String json = "{\n" +
" \"name\": \"John Doe\",\n" +
" \"age\": 30,\n" +
" \"city\": \"New York\",\n" +
" \"hobbies\": [\n" +
" \"reading\",\n" +
" \"swimming\",\n" +
" \"hiking\"\n" +
" ]\n" +
"}";
This method is not only error-prone but also hard to read and maintain. It's also tedious to write and modify, especially for larger strings. Also, it doesn't preserve the original formatting of the JSON structure.
The Solution: Java Text Blocks
Introduced as a preview feature in Java 13 and standardized in Java 15, Java Text Blocks provide a clean and intuitive way to work with multiline strings. Let's rewrite our JSON example using a Text Block:
String json = """
{
"name": "John Doe",
"age": 30,
"city": "New York",
"hobbies": [
"reading",
"swimming",
"hiking"
]
}
""";
Much better, right? The Text Block preserves the formatting, eliminates the need for most escape characters and significantly improves readability. With this, you can now handle multiline strings more elegantly.
How Java Text Blocks Work
Text Blocks are denoted by triple quotes ("""). Here are the key features:
- Opening delimiter: The opening """ must be followed by a line break.
- Closing delimiter: The closing """ can be on its own line or at the end of the last line of text content.
- Indentation: The compiler automatically removes the common whitespace prefix from each line, allowing you to indent the Text Block in your code without affecting the resulting string.
- No escape characters needed: Most special characters don't need to be escaped within a Text Block.
- Implicit line joins: Line breaks in the Text Block are preserved in the resulting string.
Why You Should Care
- Improved readability: Text Blocks clean up your code, making it easier to understand, especially when dealing with large multiline strings.
- Reduced errors: By eliminating the need for most escape characters and manual line breaks, Text Blocks reduce the likelihood of syntax errors.
- Better maintainability: Updating multiline strings becomes much easier, as you can directly edit the content without worrying about string concatenation or escape characters.
- Preserved formatting: Text Blocks help you to visually represent the desired output in your code, making it easier to spot formatting issues.
Advanced Features
Text Blocks come with some advanced features that provide even more flexibility. These are string interpolation and line control.
String interpolation
You can use the String.format() method or the newer formatted() method for string interpolation:
String name = "Alice";
int age = 30;
String message = """
Hello, %s!
You are %d years old.
""".formatted(name, age);
Escaping the closing delimiter
If you need to include """ in your Text Block, you can escape it with a backslash:
String code = """
String text = \"""
Hello, World!
\""";
""";
Controlling new lines
You can use \\ at the end of a line to suppress the following new line:
String singleLine = """
This is actually \
a single line of text.
""";
Caveats
While Text Blocks offer a a powerful, neat feature, there are a few things to keep in mind:
- Trailing whitespace: Be careful with trailing whitespace, as it's preserved in the resulting string.
- Indentation sensitivity: The indentation of the closing """ determines the common whitespace prefix that's removed from each line.
- Java version compatibility: Text Blocks are only available in Java 15 and later, so they may not be available for use in projects that need to maintain compatibility with earlier Java versions.
Conclusions
Java Text Blocks offer a clean, simple and intuitive solution for working with multiline strings. They improve code readability, reduce errors and make it easier to maintain complex string literals. Whether you're working with SQL, JSON, HTML or any other multiline text format, Text Blocks can significantly simplify your code.
Next time you find yourself wrestling with concatenated strings (is there anyone who hasn’t been there before?) and escape characters, remember that Text Blocks are here to make your life easier. Give them a try and enjoy the cleaner, more maintainable code they provide!
Happy Nugget Friday and Happy Coding!
Related Posts
10 Ways Jakarta EE 11 Modernizes Enterprise Java Development
Published on 10 Feb 2025
by Luqman Saeed
0 Comments
10 Reasons Why Java is the Future of Enterprise App Development in 2025
Published on 03 Feb 2025
by Luqman Saeed
0 Comments