Did You Know? Asynchronous REST Requests and Responses with Java EE and MicroProfile
Originally published on 04 Jan 2019
Last updated on 28 Aug 2019
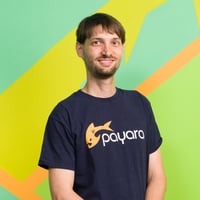
Java EE 8 fully supports asynchronous handling of REST requests and responses, on both client and server side. This is useful to optimize throughput of an application or even when adopting reactive principles. MicroProfile type-safe REST client API also supports this concept to allow you to call REST services asynchronously with a much more straightforward way with plain Java interfaces.
With the JAX-RS API in Java EE 8, you can create a method in your rest resource class that returns a CompletionStage
like this:
@GET public CompletionStage<String> hello() { return CompletableFuture.completedFuture("Hello, World!"); }
The above code returns a completed future, which isn't very helpful though. In real application, you would either return a non-completed future and complete it asynchronously later, or return a future retrieved from another asynchronous method call. In such case, the application would wait until the future is completed asynchronously in another thread before sending a response back. But meanwhile, the current thread would be released and free for processing another request.
On the client side, the JAX-RS API provides methods that call REST resources asynchronously and return CompletionStage
. This allows adding handlers that will be executed asynchronously when the response is ready and the code still stays readable. The current thread is again free to work in parallel to the asynchronous call or to be released to process other tasks. You can access the asynchronous client methods with calling the rx()
method on the client object:
WebTarget target = initRestTarget(); CompletionStage<String> asynchResult = target.request() .rx() .get(String.class)
Here, the call to get(String.class)
returns instantly and the result is handled when ready by callbacks added to asynchResult
, most probably in another thread.
Using MicroProfile REST client, the above code can be rewritten in a more natural way to Java developers as an interface:
@RegisterRestClient public interface HelloService { @GET CompletionStage<String> hello(); }
which can in turn be called like this:
HelloService service = RestClientBuilder.newBuilder() .build(HelloService.class); CompletionStage<String> asynchResult = service.hello();
It's also possible to inject an instance of HelloService
interface, so instead of writing the first line in the example above, you can get the service object like this:
@Inject @RestClient HelloService service;
All the above code is supported since Payara Server or Payara Micro version 5.183, which can run both Java EE 8 and MicroProfile 2 applications.
You can find a complete example in the Payara Examples repository.
Related Posts
A Preview of Jakarta REST (JAX-RS) 4.0 in Jakarta EE 11
Published on 13 Nov 2024
by Luqman Saeed
0 Comments
The latest version of Jakarta REST (formerly Java API for RESTful Web Services, aka JAX-RS), Jakarta REST 4.0, will bring some notable improvements and changes as part of Jakarta EE 11. This release focuses on modernizing the specification by ...
Moving Beyond GlassFish - Here's Why and How
Published on 11 Nov 2024
by Chiara Civardi
0 Comments
If you’re still managing Java applications on GlassFish middleware, you might be hitting some roadblocks, such as difficulties with automation, lack of integrated monitoring and official support and limited abilities with modern tools. However, ...